With Python E.D (Eternal Dory) Course, we’ll learn Python by analyzing the codes. We see the codes first, then learn their meanings and functions. It’s better to see things in action!
In this chapter 1, we will embark on an exploratory journey through the foundational concepts of Python, delving into variables, strings, and methods, each of which plays a pivotal role in crafting efficient and effective Python programs.
Table of Contents: 1. Variables 2. Strings 3. Methods 4. Strings’ common methods Getting Started Quick Summary: 1. Install the environment: Download and install (remember to check “Add Python to PATH”) latest Python version from https://www.python.org/downloads/. 2. Install an editor. I use Visual Studio Code (VS Code) for this course. Download and install from https://code.visualstudio.com/download 3. In VS Code > Extensions (on left bar) > Search and install Python extension (by Microsoft) 4. Use VS Code: File > New > Python file. Write codes in the window. Save file as *.py format. Run Python using the play button at top right corner. |
;
) to separate multiple statements on a single line, though this practice is generally discouraged for the sake of readability.Write and run this code in VS Code: planet_name = “earth” print(planet_name.upper( )) # Print the uppercase version of the string Output: EARTH |
1. Variables
planet_name is a variable in Python.
A variable is a name that refers to location in memory where a value is stored. Unlike some programming languages, Python does not require you to declare a variable explicitly before using it. Instead, a variable is created the moment you first assign a value to it.
Variable can hold values of different types, such as numbers (integers, floats), strings, lists, dictionaries, tuples, and more.
Python is a dynamically typed language, which means you do not need to declare the type of the variable when you created one.
*Variables naming convention: contain only letter, number, underscore. Letter and underscore and be at the beginning of a variable name, not number. Short, but should be descriptive. planet_name is better than just p, while the_planet_name is too long and unnecessary. Short and descriptive names maintain the balance between readability and efficiency. Avoid Python keywords.
# Print the uppercase version of the string is a comment in Python. Comments are lines of text in the code that are ignored by the interpreter, used to annotate the code with explanations or notes for the developer.
They are denoted by a #
symbol at the beginning of the line for single-line comments, while multi-line comments can be created by enclosing text within triple quotes ('''
or """
), although the latter is technically a multi-line string.
2. STRINGS
“Earth” is a string.
Strings are sequences of characters used to store and represent text-based information.
Strings are enclosed in quotes; you can use either single quotes ('
) or double quotes ("
), and Python treats them the same way. For strings that span multiple lines, you can use triple quotes ('''
or """
).
Try this code: multi_line_string = ” ” ” Before you know it you are already writing another line of code. Wonderful!” ” “ print(multi_line_string) |
The print()
function in Python is a built-in function that sends data to the standard output device (usually your screen). It’s one of the most commonly used functions for debugging and displaying information to the user.
Basic usage of print(): print(“Hi everyone, welcome to Eternal Dory!”) print(2024) print(“This is “, 2024, ” the year of dragon.”) |
3. methods
upper( ) is a method in Python.
In Python, a method is a function that is associated with an object and can access and modify the object’s state.
Method can be built-in (like upper( )), or user-defined (see example below).
Methods are invoked on an object using the dot notation. This means you write the name of the object, followed by a dot (.
), and then the method name with parentheses. If the method takes arguments, the arguments are placed inside the parentheses.
An example of user-defined class (will be explained in later chapters) and method. Try it yourself in VS Code. (*Notice: in the __init__ method, there are actually 2 underscores “_” must be typed next to each other, both before and after “init”; visually it would look like this: _ _init_ _) # define a new class named Superhero # __init__ is a special method, called a constructor, used for initializing new objects # shout is a simple method that take no argument (other than self) and prints a message when called class Superhero: —–def __init__ (self, nickname, catchphrase): ———-self.nickname = nickname ———-self.catchphrase = catchphrase —–def shout(self): ———-print(self.catchphrase) my_hero = Superhero(“Batman”, “I’m the Goddamn Batman.”) my_hero.shout() Output: I’m the Goddamn Batman. |
4. STRINGS’ COMMON METHODS
Here’s a list of strings’ common methods, along with an example that incorporates all of them:
upper()
: Converts all lowercase letters in a string to uppercase.lower()
: Converts all uppercase letters in a string to lowercase.title()
: Converts the first character of each word to uppercase and the rest to lowercase.capitalize()
: Converts the first character of the string to uppercase and the rest to lowercase.f-strings
: Formatted string literals that allow including expressions inside string literals using{}
(see the f-strings only examples table below).\t
: Represents a tab character that adds horizontal spacing to your output.\n
: Represents a newline character that breaks the line and moves the cursor to the beginning of the next line.strip()
: Removes leading and trailing whitespaces from the string.rstrip()
: Removes trailing whitespaces (spaces at the end) from the string.lstrip()
: Removes leading whitespaces (spaces at the beginning) from the string.removeprefix(str)
: (Python 3.9 and later) Removes the specified prefix from the start of the string, if present.
f-strings only examples: name = “Alice” age = 30 message = f”My name is {name} and I am {age} years old.” print(message) #Output: My name is Alice and I am 30 years old. a = 5 b = 10 print(f”Five plus ten is {a + b}.”) # Output: Five plus ten is 15. print(f”Curly braces: {{ and }}.”) # Output: Curly braces: { and }. name = “ALICE” print(f”Lowercased name: {name.lower()}.”) #Output: Lowercased name: alice. |
Try this code, observe the output of each print() function: #Define a string, notice there are whitespaces at the beginning and the end original_string = ” Hello, Python World! “ #Use various string methods print(original_string.upper()) # Uppercase print(original_string.lower()) # Lowercase print(original_string.title()) # Title Case print(original_string.capitalize()) # Capitalize first letter #Demonstrate f-string with tab and newline name = “Python” version = 3.9 print(f”\nName:\t{name}\nVersion:\t{version}\n”) # f-strings, \t, \n #Strip methods print(original_string.strip()) # Remove leading and trailing spaces print(original_string.rstrip()) # Remove trailing spaces print(original_string.lstrip()) # Remove leading spaces #Remove prefix (Python 3.9+) greeting = “Hello, Dory!” print(greeting.removeprefix(“Hello, “)) # Remove “Hello, ” prefix |
END OF CHAPTER 1
Learn other Python lessons at: https://eternaldory.com/category/knowledge/python/
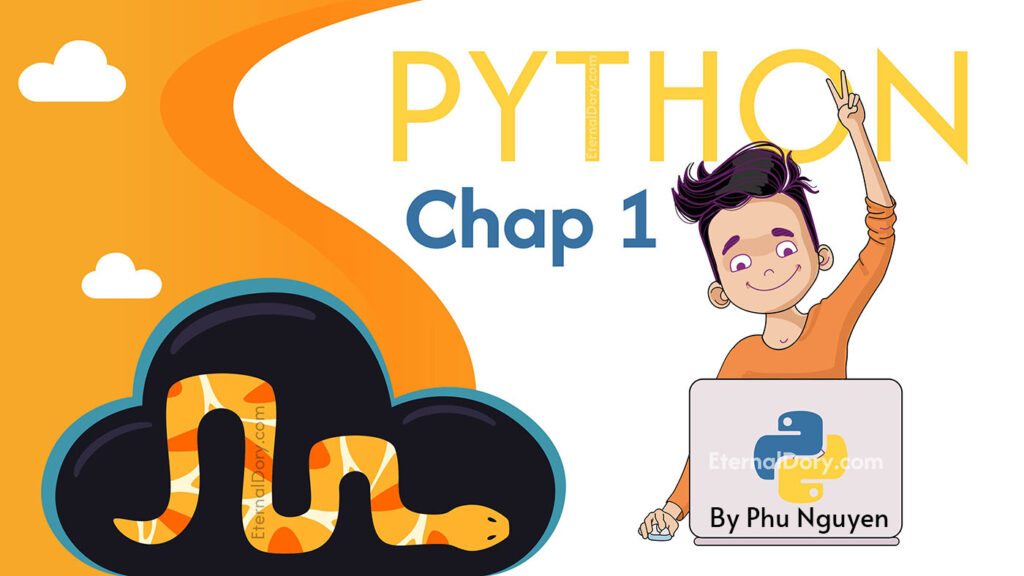
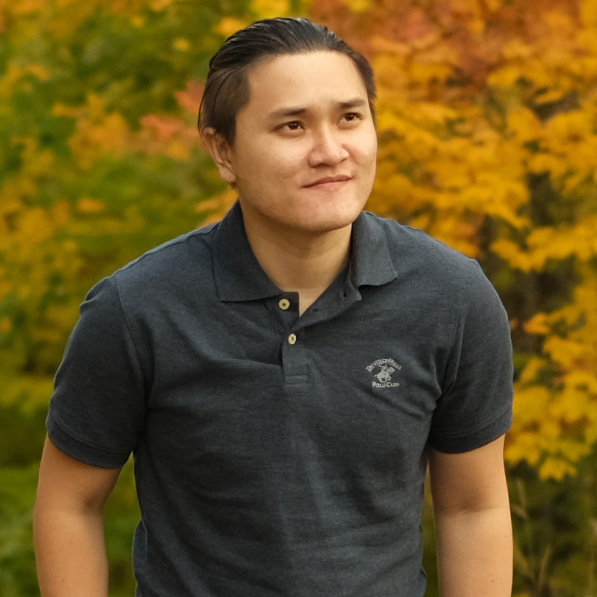
Phu is the Head Writer at Eternal Dory, where his pen dives into the latest global trends and news in knowledge, graphic design, metaphysics, and entertainment. He has a knack for highlighting the fun aspects of his subjects, lightening the mood. Phu’s academic background is in Graphic Design, focusing on Animation and Motion Graphics.